It is often useful to pass certain parameters to a python script. For example: if you need a batch job to run on a desired queue, if want to specify desired memory allocation, or if you simply need to define the name of a file being generated by the script. A quick and easy solution to handle changing parameters is to include code that accepts user-defined input.
Example code: command-line-arguments-example.py
import argparse
def parse_args():
parser = argparse.ArgumentParser(add_help=False)
# arguments that provide script information
parser.add_argument(
'-v',
'--version',
action='version',
version='%(prog)s 1.0',
help="Show program's version number and exit."
)
parser.add_argument(
'-h',
'--help',
action='help',
default=argparse.SUPPRESS,
help='Show this help message and exit.'
)
# required arguments
parser.add_argument(
"-m",
"--memory",
type=int,
metavar="",
required=True,
help="""Memory allocated to job."""
)
parser.add_argument(
"-q",
"--jobQueue",
type=str,
metavar="",
required=True,
choices=["analysis-queue-1", "analysis-queue-2"],
help="""Batch queue. Select from: analysis-queue-1, analysis-queue-2"""
)
# optional argument with default value set
parser.add_argument(
"-n",
"--jobName",
type=str,
metavar="",
default="analysis-job",
help="""AWS Batch job name. Default value: analysis-job."""
)
return parser.parse_args()
def main():
args = parse_args()
mem = args.memory
queue= args.jobQueue
jobName = args.jobName
print("Memory:",mem)
print("Queue:",queue)
print("Job Name:",jobName)
if __name__ == "__main__":
main()
Example usage:

Let’s break down the elements:
- Import the
argparse
module, which allows users to input data, provides help messages, and shows errors if arguments are invalid.
import argparse
- Provide a version for your script. Generally, this is a good programming practice. If you need guidance for versioning, visit this blog post. Note that a ‘help’ message in included for this argument all subsequent arguments. Use this to describe the basic function of the argument.
parser.add_argument(
'-v',
'--version',
action='version',
version='%(prog)s 1.0',
help="Show program's version number and exit."
)

- Describe the purpose of the python script. What does this program accomplish? This text is shown when the user enters help mode. The help message for each command-line argument is also displayed.
parser.add_argument(
'-h',
'--help',
action='help',
default=argparse.SUPPRESS,
help='Show this help message and exit.'
)
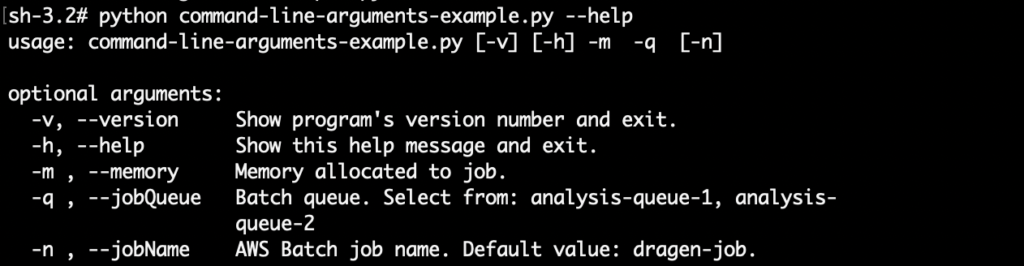
- Add required arguments with
required
set toTrue
. These arguments can passed to the script using either the shorthand or long version, ie.-m 400
and--memory 400
are programmatically equivalent. Include the variable type, such asint
orstr
. Note that the job name argument only accepts 2 values. Use this feature to control what users can enter for arguments. Finally,metavar
is used a placeholder for the help message display.
# required argument
parser.add_argument(
"-m",
"--memory",
type=int,
metavar="",
required=True,
help="""Memory allocated to job.""",
)
parser.add_argument(
"-q",
"--jobQueue",
type=str,
metavar="",
required=True,
choices=["analysis-queue-1", "analysis-queue-2"],
help="""Batch queue. Select from: analysis-queue-1, analysis-queue-2"""
)
- Add optional requirements and set a default value. Notice that the
required
parameter is not set because the default value isFalse
.
parser.add_argument(
"-n",
"--jobName",
type=str,
metavar="",
default="analysis-job",
help="""AWS Batch job name. Default value: analysis-job."""
)
- Close out the parse_args() function by returning all of the arguments.
return parser.parse_args()
- Access user-defined command input in your script by calling the
parse_args
function.
def main():
args = parse_args()
mem = args.memory
queue= args.jobQueue
jobName = args.jobName
print("Memory:",mem)
print("Queue:",queue)
print("Job Name:",jobName)
if __name__ == "__main__":
main()
Additional Helpful Resources:
Click here to report typos.